94.Binary Tree In order Traversal
Given the root
of a binary tree, return the in order traversal of its nodes’ values.
Example 1:
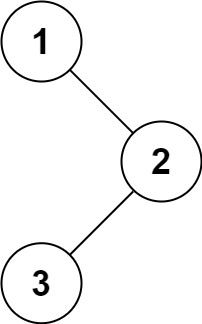
1 2
| Input: root = [1,null,2,3] Output: [1,3,2]
|
Example 2:
1 2
| Input: root = [] Output: []
|
Example 3:
1 2
| Input: root = [1] Output: [1]
|
递归法:
时间复杂度:O(n)
空间复杂度:O(n)
1 2 3 4 5 6 7 8 9 10 11 12 13
| void inorder(TreeNode* root, vector<int>& res) { if (!root) { return; } inorder(root->left, res); res.push_back(root->val); inorder(root->right, res); } vector<int> inorderTraversal(TreeNode* root) { vector<int> res; inorder(root, res); return res; }
|
迭代法:
时间复杂度:O(n)
空间复杂度:O(n)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| vector<int> inorderTraversal(TreeNode* root) { vector<int> res; stack<TreeNode*> stk; while (root != nullptr || !stk.empty()) { while (root != nullptr) { stk.push(root); root = root->left; } root = stk.top(); stk.pop(); res.push_back(root->val); root = root->right; } return res; }
|
Morris 中序遍历:
时间复杂度:O(n)
空间复杂度:O(1)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| vector<int> inorderTraversal(TreeNode* root) { vector<int> res; TreeNode *predecessor = nullptr;
while (root != nullptr) { if (root->left != nullptr) { predecessor = root->left; while (predecessor->right != nullptr && predecessor->right != root) { predecessor = predecessor->right; }
if (predecessor->right == nullptr) { predecessor->right = root; root = root->left; } else { res.push_back(root->val); predecessor->right = nullptr; root = root->right; } } else { res.push_back(root->val); root = root->right; } } return res; }
|