144.Binary Tree Preorder Traversal
Given the root
of a binary tree, return the preorder traversal of its nodes’ values.
Example 1:
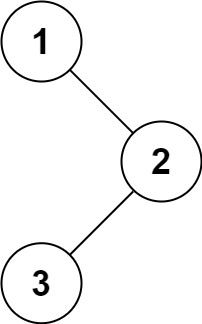
1 2
| Input: root = [1,null,2,3] Output: [1,2,3]
|
Example 2:
1 2
| Input: root = [] Output: []
|
Example 3:
1 2
| Input: root = [1] Output: [1]
|
时间复杂度:O(n)
空间复杂度:O(n)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class Solution { public: void preorder(TreeNode *root, vector<int> &ans_v) { if (root == nullptr)return; else { ans_v.push_back(root->val); preorder(root->left,ans_v); preorder(root->right,ans_v); } } vector<int> preorderTraversal(TreeNode* root) { vector<int> ans_v; preorder(root,ans_v); return ans_v; } };
|
迭代法:
时间复杂度:O(n)
空间复杂度:O(n)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| class Solution { public: vector<int> preorderTraversal(TreeNode* root) { vector<int> res; if (root == nullptr) { return res; }
stack<TreeNode*> stk; TreeNode* node = root; while (!stk.empty() || node != nullptr) { while (node != nullptr) { res.emplace_back(node->val); stk.emplace(node); node = node->left; } node = stk.top(); stk.pop(); node = node->right; } return res; } };
|
Morris 遍历:
时间复杂度:O(n)
空间复杂度:O(1)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| class Solution { public: vector<int> preorderTraversal(TreeNode *root) { vector<int> res; if (root == nullptr) { return res; }
TreeNode *p1 = root, *p2 = nullptr;
while (p1 != nullptr) { p2 = p1->left; if (p2 != nullptr) { while (p2->right != nullptr && p2->right != p1) { p2 = p2->right; } if (p2->right == nullptr) { res.emplace_back(p1->val); p2->right = p1; p1 = p1->left; continue; } else { p2->right = nullptr; } } else { res.emplace_back(p1->val); } p1 = p1->right; } return res; } };
|