145.Binary Tree Postorder Traversal
Given the root
of a binary tree, return the postorder traversal of its nodes’ values.
Example 1:
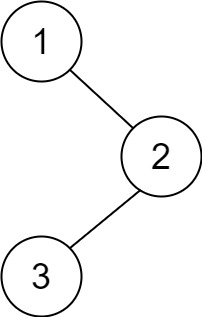
1 2
| Input: root = [1,null,2,3] Output: [3,2,1]
|
Example 2:
1 2
| Input: root = [] Output: []
|
Example 3:
1 2
| Input: root = [1] Output: [1]
|
递归法:
时间复杂度:O(n)
空间复杂度:O(n)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class Solution { public: void postorder(TreeNode* root, vector<int> &ans_v) { if (root == nullptr)return; else { postorder(root->left,ans_v); postorder(root->right,ans_v); ans_v.push_back(root->val); } } vector<int> postorderTraversal(TreeNode* root) { vector<int> ans_v; postorder(root,ans_v); return ans_v; } };
|
迭代法:
时间复杂度:O(n)
空间复杂度:O(n)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| class Solution { public: vector<int> postorderTraversal(TreeNode *root) { vector<int> res; if (root == nullptr) { return res; }
stack<TreeNode *> stk; TreeNode *prev = nullptr; while (root != nullptr || !stk.empty()) { while (root != nullptr) { stk.emplace(root); root = root->left; } root = stk.top(); stk.pop(); if (root->right == nullptr || root->right == prev) { res.emplace_back(root->val); prev = root; root = nullptr; } else { stk.emplace(root); root = root->right; } } return res; } };
|
Morris遍历:
时间复杂度:O(n)
空间复杂度:O(1)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| class Solution { public: void addPath(vector<int> &vec, TreeNode *node) { int count = 0; while (node != nullptr) { ++count; vec.emplace_back(node->val); node = node->right; } reverse(vec.end() - count, vec.end()); }
vector<int> postorderTraversal(TreeNode *root) { vector<int> res; if (root == nullptr) { return res; }
TreeNode *p1 = root, *p2 = nullptr;
while (p1 != nullptr) { p2 = p1->left; if (p2 != nullptr) { while (p2->right != nullptr && p2->right != p1) { p2 = p2->right; } if (p2->right == nullptr) { p2->right = p1; p1 = p1->left; continue; } else { p2->right = nullptr; addPath(res, p1->left); } } p1 = p1->right; } addPath(res, root); return res; } };
|