203.Remove Linked List Elements
Given the head
of a linked list and an integer val
, remove all the nodes of the linked list that has Node.val == val
, and return the new head.
Example 1:
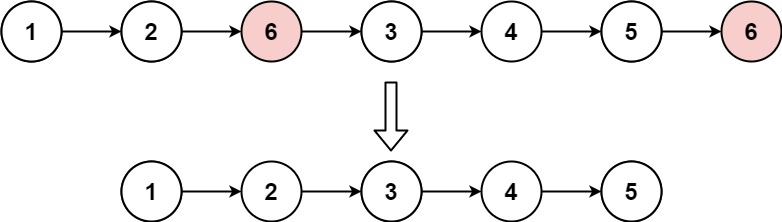
1 2
| Input: head = [1,2,6,3,4,5,6], val = 6 Output: [1,2,3,4,5]
|
Example 2:
1 2
| Input: head = [], val = 1 Output: []
|
Example 3:
1 2
| Input: head = [7,7,7,7], val = 7 Output: []
|
迭代法:
时间复杂度:O(n)
空间复杂度:O(1)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| class Solution { public: ListNode* removeElements(ListNode* head, int val) { while (head != NULL && head->val == val)head = head->next; ListNode* t = head; ListNode* in = head; while (t != NULL) { if (t->val == val) { in->next = t->next; t = t->next; continue; } in = t; t = t->next; } return head; } };
|
优化:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| class Solution { public: ListNode* removeElements(ListNode* head, int val) { ListNode* dummyHead = new ListNode(0, head); ListNode* temp = dummyHead; while (temp->next != NULL) { if (temp->next->val == val) { temp->next = temp->next->next; } else { temp = temp->next; } } return dummyHead->next; } };
|
递归法:
时间复杂度:O(n)
空间复杂度:O(n)
1 2 3 4 5 6 7 8 9 10
| class Solution { public: ListNode* removeElements(ListNode* head, int val) { if (head == nullptr) { return head; } head->next = removeElements(head->next, val); return head->val == val ? head->next : head; } };
|