257.Binary Tree Paths
Given the root
of a binary tree, return all root-to-leaf paths in any order.
A leaf is a node with no children.
Example 1:
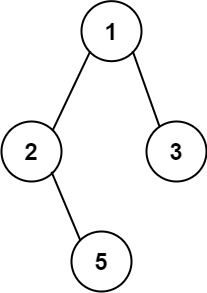
1 2
| Input: root = [1,2,3,null,5] Output: ["1->2->5","1->3"]
|
Example 2:
1 2
| Input: root = [1] Output: ["1"]
|
深度优先遍历:
时间复杂度:O(n2)
空间复杂度:O(n2)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| class Solution { public: vector<string> ret; void next(TreeNode* root, string path) { if (root != nullptr) { path += to_string(root->val); if (root->left != nullptr || root->right != nullptr) { path += "->"; next(root->left, path); next(root->right, path); } else { ret.push_back(path); } } } vector<string> binaryTreePaths(TreeNode* root) { next(root,""); return ret; } };
|
广度优先遍历:
时间复杂度:O(n2)
空间复杂度:O(n2)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| class Solution { public: vector<string> binaryTreePaths(TreeNode* root) { vector<string> paths; if (root == nullptr) { return paths; } queue<TreeNode*> node_queue; queue<string> path_queue;
node_queue.push(root); path_queue.push(to_string(root->val));
while (!node_queue.empty()) { TreeNode* node = node_queue.front(); string path = path_queue.front(); node_queue.pop(); path_queue.pop();
if (node->left == nullptr && node->right == nullptr) { paths.push_back(path); } else { if (node->left != nullptr) { node_queue.push(node->left); path_queue.push(path + "->" + to_string(node->left->val)); }
if (node->right != nullptr) { node_queue.push(node->right); path_queue.push(path + "->" + to_string(node->right->val)); } } } return paths; } };
|